Project Overview
Terra Got Away is a singleplayer sprint-survival game. The player's goal is to find their way through multiple levels without running out of heat or water in order to complete the game. The game was created during the 2019 Global Game Jam event in a team of five.
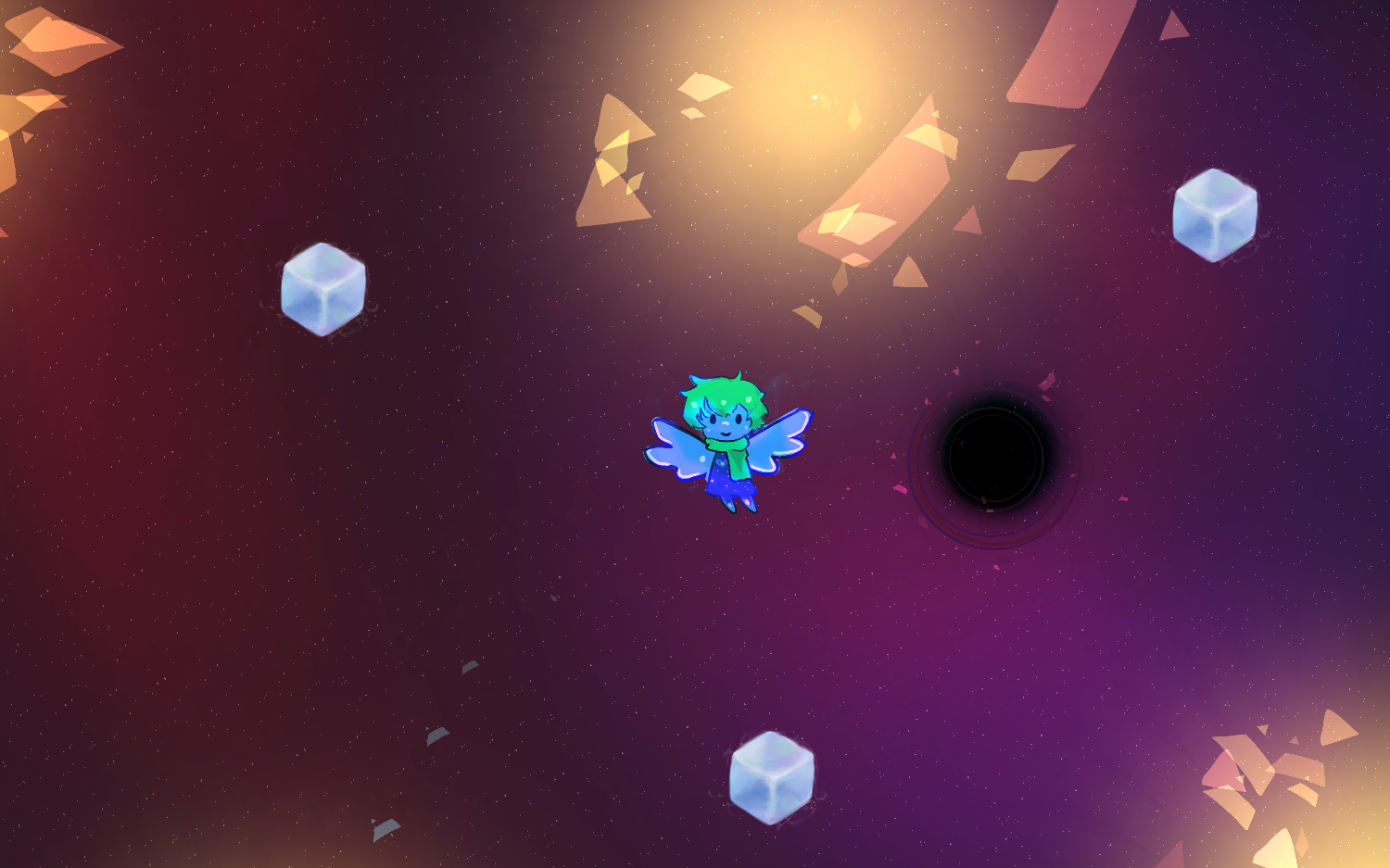
The Recursive World
The world's recursivity has been achieved by orienting the 2D elements of the game around a 3D sphere. A drawback to this method is that other objects will appear to have clipped the "background" sphere and suffer from innacurate collisions due to their angle and positioning relative to each other. To fix those issues, the objects align with the player when on the same side of the sphere.
public class Player : MonoBehaviour
{
[SerializeField] float moveSpeed = 15;
[SerializeField] bool randomRotStart = true;
void Start()
{
if (randomRotStart)
{
transform.eulerAngles = new Vector3(0, 0, Random.Range(0, 360));
}
}
void Update()
{
float up = Input.GetAxis("Vertical");
float right = Input.GetAxis("Horizontal");
Vector3 movement = transform.rotation * new Vector3(-up, right);
transform.RotateAround(Vector3.zero, -movement, moveSpeed * Time.deltaTime);
}
}
[ExecuteInEditMode]
public class AlignWithSphere : MonoBehaviour
{
public Vector3 centerRot = Vector3.forward;
Transform player;
void Start()
{
player = GameObject.Find("Player").transform;
}
void Update()
{
transform.position = Quaternion.Euler(centerRot) * Vector3.forward * 55;
transform.eulerAngles = centerRot;
if (player)
{
transform.rotation = player.transform.rotation;
Vector3 toPlayer = Vector3.Normalize(player.position);
float dotDistance = Vector3.Dot(toPlayer, transform.position);
if (dotDistance > 0)
{
float difference = player.position.magnitude - dotDistance;
Vector3 fixedPosition = transform.position + (toPlayer * difference);
transform.position = fixedPosition;
}
}
}
}